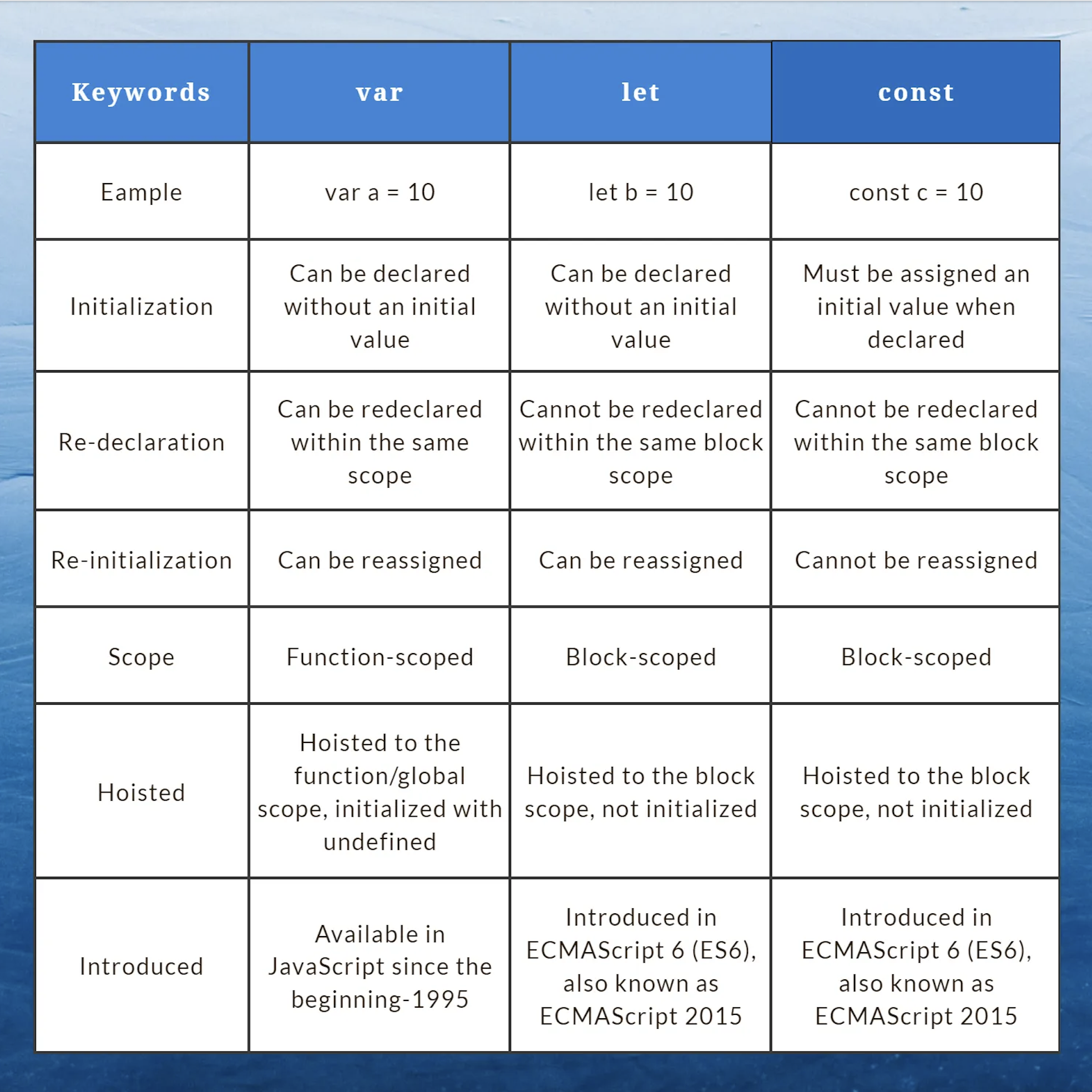
Variable Declarations: var, let and const
var
var is function scoped
var is globally scoped when declared outside of a function.
function doWhatEver() {
if (2>1) {
var foo = "hello"
}
console.log(foo)
}
doWhatEver()
console.log(foo)
// Output: hello
// Cant find variable: foo
Primitive Variable Types
//Numbers
var num = 42;
var meaning = "The meaning of life is ";
var life = meaning + num;
var otherNum = "15";
var num = 10;
var whaaa = otherNum - num;
var likeAnActualNum = parseInt(otherNum, 10); //15
var floaty = "1.5";
var likeAnActualDecimal = parseFloat(floaty); //1.5
//Strings
var foo = "There is no spoon";
var bar = "No, \"really\", " + foo; //No, "really", There is no spoon
var barBetter = "No, \"really\", " + foo.toLowerCase();
// Undefined
var a; // has value of undefined
//console.log(b); // Uncaught ReferenceError: b is not defined
if(b){
//console.log('a b!');
}
When a variable is declared but not assigned a value then it is undefined.
This might be ... the app will get the value later.
// null
var a = null; // initialised but with no value
//console.log(a); // null
Null gets assigned to variables ... it indicates an desired missing of a value.